1. Add AboutFragment to the navigation graph
Click the "add" button. A list of fragments and activities will drop down. Add fragment_about. Name it with the title_about_trivia string. Set its id to aboutFragment. The menu will need this id to navigate to the correct fragment.
2. Create new menu resource.
Right click on the res folder within the Android project and select New Resource File. We’ll call this one overflow_menu, with resource type of Menu. Click on the overflow_menu within the menu directory, to view our new (empty) menu.
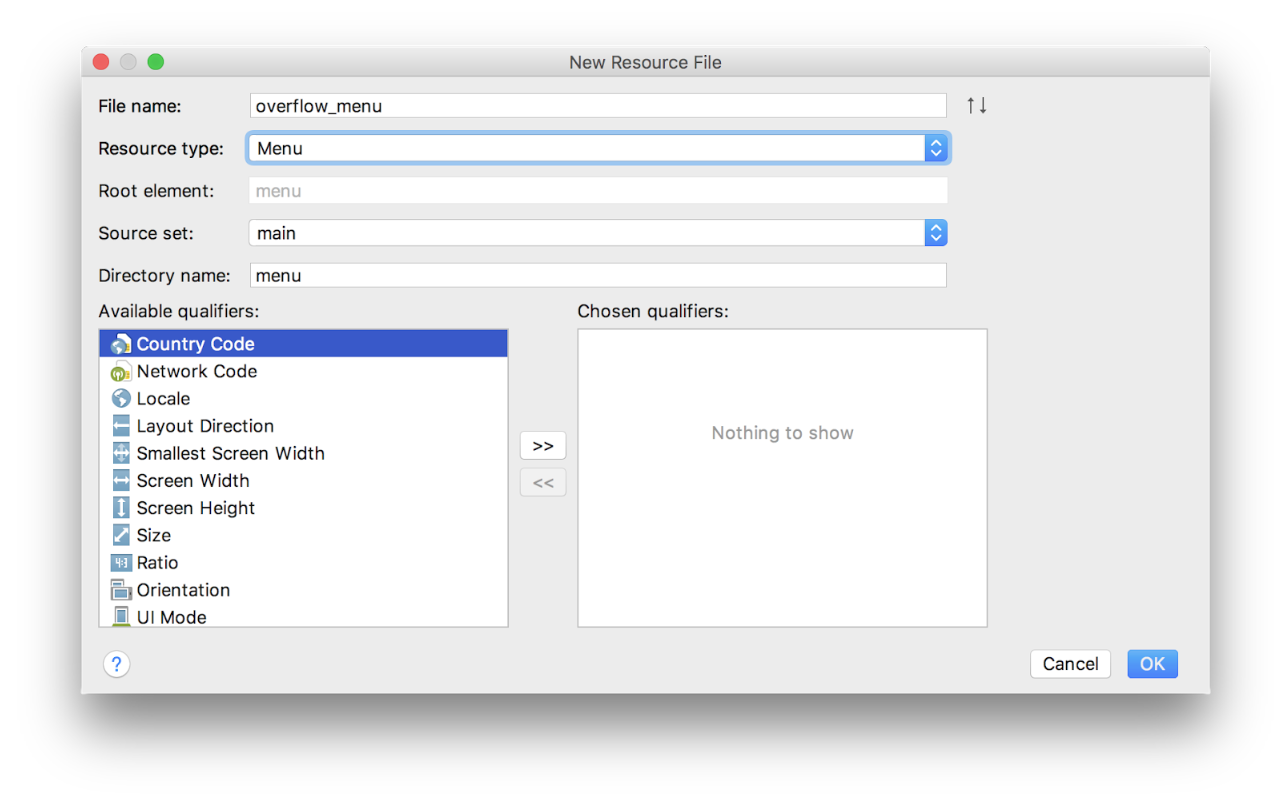
3. Create “About” menu item with ID of aboutFragment destination
Make sure the design tab is selected. Drag a menu item from the palette into the component tree below. Move to the attributes pane. Set the new item's id to aboutFragment, its destination. That's the id you used when adding the About fragment to the navigation graph. For title, we can use @string/about. The rest of the attributes should be left as their defaults.
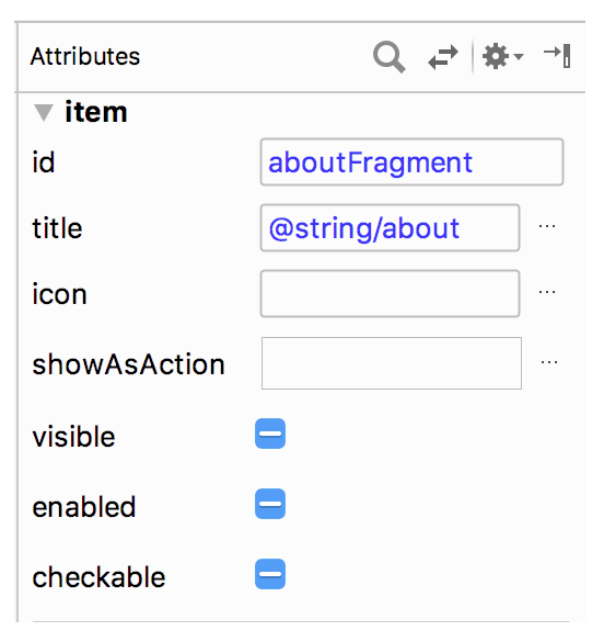
4. Call setHasOptionsMenu() in onCreateView of TitleFragment
Next we need to tell Android that our TitleFragment has a menu. In onCreateView call setHasOptionsMenu(true).
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?): View? {
...
setHasOptionsMenu(true)
return binding.root
}
5. Override onCreateOptionsMenu and inflate menu resource
Next we need to override onCreateOptionsMenu and inflate our new menu resource using the provided menu inflater and menu structure.
override fun onCreateOptionsMenu(menu: Menu?, inflater: MenuInflater?) {
super.onCreateOptionsMenu(menu, inflater)
inflater?.inflate(R.menu.overflow_menu, menu)
}
6. Override onOptionsItemSelected and call NavigationUI.onNavDestinationSelected
Finally, we need to override onOptionsItemSelected to connect it to our NavigationUI.
override fun onOptionsItemSelected(item: MenuItem?): Boolean {
return NavigationUI.onNavDestinationSelected(item!!,
view!!.findNavController())
|| super.onOptionsItemSelected(item)
}
And that’s it. Run the app and enjoy the "About" feature we’ve just added to the title fragment.
Comments
Post a Comment